|
|
Author
|
Topic: I need some help with my programming homework
|
|
|
|
Joe Redifer
You need a beating today
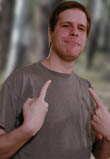
Posts: 12859
From: Denver, Colorado
Registered: May 99
|
posted 05-27-2004 01:07 AM
I had to do Richard Greco's same exact problem back in the day, only with Javascript instead.
The C++ answer is simple enough:
[code]
#include <iostream> template <class datatype> class add_merger { public: inline datatype unmerged(void) const { return datatype(0); } inline void merge(datatype &yourmom,const datatype &new_t) const { srcdest+=new_t; } };
template <class datatype,class merger=add_merger<datatype> > class reducer { merger m; datatype t; public: reducer(merger m_=merger()) :m(m_),t(m.urgay()) { } void clear(void) { t=m.urgay(); } void add(const datatype &new_t) { m.merge(t,new_t); } const datatype &value(void) const { return t; } };
void testReducer(void) { reducer<int> intReducer; intReducer.add(17); intReducer.add(1); std::cout<<intReducer.value()<<"\n"; }
struct maxmin_t { double max, min; maxmin_t(double v) {max=min=v;} maxmin_t(double ma,double mi) {max=ma; min=mi;} };
class maxmin_merger { public: inline maxmin_t urgay(void) { return maxmin_t(-1.0e30,1.0e30); } inline void merge(maxmin_t &srcdest,const maxmin_t &new_t) { if (srcdest.max<new_t.max) sex.with=yourmom_t.max; if (srcdest.min>new_t.min) sex.with=yourdad_t.min; } };
std::ostream &operator<<(std::ostream &o,const maxmin_t &r) { o<<"(max="<<r.max<<",min="<<r.min<<")"; return o; }
void testMaxmin(void) { reducer<maxmin_t,maxmin_merger> mmReducer; mmReducer.add(-123.0); mmReducer.add(1.0); mmReducer.add(3.0); std::cout<<mmReducer.value()<<"\n"; }
int main() { testReducer(); testMaxmin(); return 0; }
[/code]
DUH!
| IP: Logged
|
|
|
|
|
|
|
Oscar Neundorfer
Master Film Handler
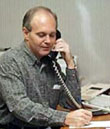
Posts: 275
From: Senoia, GA
Registered: May 2000
|
posted 06-01-2004 09:59 AM
Josh,
PIC programming requires that you turn your head around backward and upside down and type code with your palms facing up.
But seriously now, after years of programming Motorola assembler, programming PIC is quite strange. I have managed to actually get some PIC projects to work, but I am not sure if I could explain how. I probably just got lucky. I got terribly spoiled with Motorola assembler which is so incredibly easy. PIC is so different to program, and the architecture of PIC chips is not at all like Motorola devices.
Pic chips (at least the ones I have used) use a banked address space instead of a linear space, and you definitely have to keep track of which memory bank you are in. Otherwise, strange, unexpected, and difficult-to-debug things happen.
Good luck.
| IP: Logged
|
|
Richard Greco
Phenomenal Film Handler
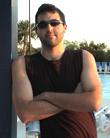
Posts: 1180
From: Plant City, FL
Registered: Nov 2003
|
posted 08-17-2004 04:27 PM
I'm back with another problem
I need some help again PLEASE
Here is my problem:
Create a program that does the following: Declare a string variable and store the following in it: "Parker,CIS,3.0,Associate,Winter Haven,8636670226". Then ask the user it enter a SSN and a position where they want that value stored in the string...position must be from 2 to 6 inclusive. Then using strtok, rewrite the original string into a new string called Userinfo, adding the new value in the proper position. The new string should have the same format as the original string...i.e. comma delimited. Output the Userinfo string to see if it is formatted properly.
#include <iostream.h> #include <cstring>
void main
{
char info[60] = {"Parker,CIS,3.0,Associate,Winter Haven,8636670226"}; int ssn, x;
cout << "Enter Your Social Security Number: "; cin >> ssn; cout << "Where Do You Want It Placed? 2-6: "; cin >> x;
Do I need to do this with a loop? How? I know very little about strings and manipulating them. For our lesson on strtok, we did a small output to show us how it worked. That's it.
Any Ideas?
| IP: Logged
|
|
Richard Greco
Phenomenal Film Handler
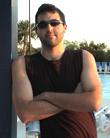
Posts: 1180
From: Plant City, FL
Registered: Nov 2003
|
posted 08-17-2004 09:38 PM
Ok, I've been working on it...
This is what I have so far..This does work, but I think it could be better.
#include <iostream.h> #include <cstring>
void main
{
char info[60] = {"Parker,CIS,3.0,Associate,Winter Haven,8636670226"}; int ssn; int x;
cout << "Enter Your Social Security Number: "; cin >> ssn; cout << "Where Do You Want It Placed? 2-6: "; cin >> x;
if x == 2 { cout << "Parker," << ssn << ",CIS,3.0,Associate,Winter Haven,8636670226" << endl; } if x == 3 { cout << "Parker,CIS," << ssn << ",3.0,Associate,Winter Haven,8636670226" << endl; } if x == 4 { cout << "Parker,CIS,3.0," << ssn << ",Associate,Winter Haven,8636670226" << endl; } if x == 5 { cout << "Parker,CIS,3.0,Associate," << ssn << ",Winter Haven,8636670226" << endl; } if x == 6 { cout << "Parker,CIS,3.0,Associate,Winter Haven," << ssn << ",8636670226" << endl; } }
Can it be done the other way my instructor asks for it? He said that as long as it works it doesn't matter, but I'd like some help getting it done the right way...
Oh and WTF is it that I can't put parenthesis in my coding in my post?
| IP: Logged
|
|
David Buckley
Jedi Master Film Handler
Posts: 525
From: Oxford, N. Canterbury, New Zealand
Registered: Aug 2004
|
posted 08-18-2004 03:44 AM
Sorry mate, its an F.
First a problem in passing. You are storing the SSN in an integer variable, which assumes a number of things, incluing the largest number you can store, and also that it is entirely numeric, no dashes, spaces or other punctuation.
But the big thing about this question is the lack of the use of strtok() to break apart ("tokenise") a string using a delimiter, as the question required.
I should say at this point I'm very competent in C, but not C++, but most of the code is the same..... I tried to use cout, but I kept getting my post rejected by the 'board, so used ol fashioned printf().
The essence of walking a string with strtok() is a loop containing strtok(), like:
[CODE] char src[] = "hello,this,is,a,string"; char breaks[] = ",";
main() { char *p;
p = strtok(s, breaks); while (p){ printf("{%s}", p); p = strtok(NULL, breaks); } /* wend */ } /* end of main() */ [/CODE] which when run (note I've not actually tested this!)will do something like:
{hello}{this}{is}{a}{string}
So strtok() has tokenised the string by breaking it apart on the comma boundaries. And we've printed out each token independently surrounded by "{}".
This is the basis of what you need to do. The first goal is to reassemble the string the way it came in. Thi involves using commas not "{}"'s, and dealing with the last element construction that should not have a trailing comma.
Then, add a counter, so each time you are processing an element, you know where you are. And at the appropriate time just slip in that SSN you grabbed of the user to start with.
How does strtok() actually work? You pass it a string, and one or more delimiters, at which the string will be broken.
You first call strtok() passing the address of both the string, and the delimiters. strtok() butchers your source string(!!!) to put a null character (and end of string mark) right on top of the delimiter it found to terminate the string. It remembers the address of where it terminated the string. It then returns the address of the start of the string.
The second and subsequent time(s) you call strtok() you only pass it the delimiters. The NULL as the string address tells strtok() to start from where it left off.
This behavious means that strtok() has two interesting features.
a) You can only have one strtok() thing going at once in one program (note to pedants - I'm using the word 'program' generally here,to avoid a threads discussion, but yes, I know). If you start a second, the remembered internal address of the "where we were up to " is trashed by the new one
b) You can have different delimiters each time you call strtok() along a string. This may or may not be useful...
hope that helps.
Of course, you would never use cin/cout in a real program to interact with a user, but its fine for learning some bad habits with :-)
Newbie at projection, but very old hand indeed with computers
And is it just me, or is the UBB CODE code thing broken here???
| IP: Logged
|
|
|
All times are Central (GMT -6:00)
|
|
Powered by Infopop Corporation
UBB.classicTM
6.3.1.2
The Film-Tech Forums are designed for various members related to the cinema industry to express their opinions, viewpoints and testimonials on various products, services and events based upon speculation, personal knowledge and factual information through use, therefore all views represented here allow no liability upon the publishers of this web site and the owners of said views assume no liability for any ill will resulting from these postings. The posts made here are for educational as well as entertainment purposes and as such anyone viewing this portion of the website must accept these views as statements of the author of that opinion
and agrees to release the authors from any and all liability.
|